In many cases, while working with lightning:datatable we got challenge to display URL which redirect to record page and also for related lookup fields and formula fields. If it’s a Salesforce Id, a lookup to record or to some other record, and we would like to display it as a link with an appropriate value. Unfortunately, <lightning:dataTable>
doesn’t have an Id
column type, and the url
type is not smart enough to realize it’s been handed a record Id and handle it.
Instead, we need to generate the URL ourselves and add it as a property of the line items in the source data. So for that one we have to use typeAttributes in column property.
Basically typeAttributes are the one of the major building blocks of <lightning:datatable>. This will help us to format the data types /output display and attaching some of actions to your data displayed in table at each cell level ,row level or at the columns level.
The typeAttributes are attached to each columns data type when your specifying the columns for your data table in your java script file. Each typeAttributes is having pre-defined set of attributes/properties basis on the data type of your column (your defining at the time of columns for table).
Hyperlink/Image Formula :
Here we have one formula field Demo Formula on Task where it will display task Priority name on click on that link it will redirect it to Task List view. So to use that formula in data table we have to do following.
Inside Column we have to set typeAttributes like below.
{
label: 'Demo Formula',
fieldName: 'DemoLink',
type: "url",
typeAttributes: {
label: {
fieldName: 'Priority'
},
target: '_blank'
}
},
//For Image formula define colums like this.
{
label: 'Status',
fieldName: 'Status',
cellAttributes: {
iconName: {
fieldName: 'statusIconName'
},
iconLabel: {
fieldName: 'statusIconLabel'
},
iconPosition: 'right'
}
}
Also inside response we have to handle it like below.
var records = response.getReturnValue();
records.forEach(function(record) {
record.Priority = record.Priority;
record.DemoLink = '/lightning/o/Task/home'; //Define here hyperlink as per formula field
//For Image you can add icons dynamically like this.
if (record.Status === 'Not Started') {
record.statusIconName = 'standard:assignment';
record.statusIconLabel = record.Status;
record.Status = '';
}
if (record.Status === 'In Progress') {
record.statusIconName = 'standard:investment_account';
record.statusIconLabel = record.Status;
record.Status = '';
}
});
component.set("v.taskList", records);
Name/Lookup/Related Field :
For any related field or lookup/Name field we have to change both display name and link functionality. For example on Task we have WhatId so we have to display Related To name and we have to set hyperlink here. So to use that field in data table we have to do following.
Inside Column we have to set typeAttributes like below.
{
label: 'Related To',
fieldName: 'WhatId',
type: 'url',
typeAttributes: {
label: {
fieldName: 'WhatName'
},
target: '_blank'
}
}
Also inside response we have to handle it like below.
var records = response.getReturnValue();
records.forEach(function(record) {
record.WhatName = record.What.Name; //Here define your value which you want to display
record.WhatId = '/' + record.WhatId ';// Here define where you want to redirect.
});
component.set("v.taskList", records);
Let’s try all together inside lightning component.
Here is component output.

Apex Class
public with sharing class TaskListController {
@AuraEnabled(cacheable=true)
public static List<Task> getTaskList() {
return [SELECT Id,Subject, ActivityDate, What.Name, WhatId, OwnerId,Owner.Name FROM Task LIMIT 10];
}
}
TaskList.cmp
<aura:component implements="force:appHostable,flexipage:availableForAllPageTypes" controller="TaskListController">
<aura:attribute type="Task[]" name="taskList"/>
<aura:attribute name="mycolumns" type="List"/>
<aura:handler name="init" value="{!this}" action="{!c.fetchTasks}"/>
<lightning:card title="Datatable Example" iconName="action:new_task">
<lightning:datatable data="{!v.taskList}"
columns="{! v.mycolumns }"
keyField="id"
hideCheckboxColumn="true"/>
</lightning:card>
</aura:component>
TaskListController.js
({
fetchTasks: function(component, event, helper) {
component.set('v.mycolumns', [{
label: 'Subject',
fieldName: 'subjectLink',
type: 'url',
typeAttributes: {
label: {
fieldName: 'Subject'
},
target: '_blank'
}
},
{
label: 'Due Date',
fieldName: 'ActivityDate',
type: "date",
typeAttributes: {
weekday: "long",
year: "numeric",
month: "long",
day: "2-digit"
}
},
{
label: 'Demo Formula',
fieldName: 'DemoLink',
type: "url",
typeAttributes: {
label: {
fieldName: 'Priority'
},
target: '_blank'
}
},
{
label: 'Related To',
fieldName: 'WhatId',
type: 'url',
typeAttributes: {
label: {
fieldName: 'WhatName'
},
target: '_blank'
}
},
{
label: 'Status',
fieldName: 'Status',
cellAttributes: {
iconName: {
fieldName: 'statusIconName'
},
iconLabel: {
fieldName: 'statusIconLabel'
},
iconPosition: 'right'
}
},
{
label: 'Assigned To',
fieldName: 'OwnerId',
type: 'url',
typeAttributes: {
label: {
fieldName: 'OwnerName'
},
target: '_blank'
}
}
]);
var action = component.get("c.getTaskList");
action.setParams({});
action.setCallback(this, function(response) {
var state = response.getState();
if (state === "SUCCESS") {
var records = response.getReturnValue();
records.forEach(function(record) {
record.WhatName = record.What.Name;
record.WhatId = '/' + record.WhatId;
record.Priority = record.Priority;
record.DemoLink = '/lightning/o/Task/home';
record.OwnerName = record.Owner.Name;
record.OwnerId = '/' + record.OwnerId;
record.subjectLink = '/' + record.Id;
if (record.Status === 'Not Started') {
record.statusIconName = 'standard:assignment';
record.statusIconLabel = record.Status;
record.Status = '';
}
if (record.Status === 'In Progress') {
record.statusIconName = 'standard:investment_account';
record.statusIconLabel = record.Status;
record.Status = '';
}
if (record.Status === 'Waiting on someone else') {
record.statusIconName = 'standard:call';
record.statusIconLabel = record.Status;
record.Status = '';
}
if (record.Status === 'Deferred') {
record.statusIconName = 'standard:first_non_empty';
record.statusIconLabel = record.Status;
record.Status = '';
}
if (record.Status === 'Completed') {
record.statusIconName = 'standard:task2';
record.statusIconLabel = record.Status;
record.Status = '';
}
});
console.log(records);
}
component.set("v.taskList", records);
});
$A.enqueueAction(action);
}
})
Live Demo :
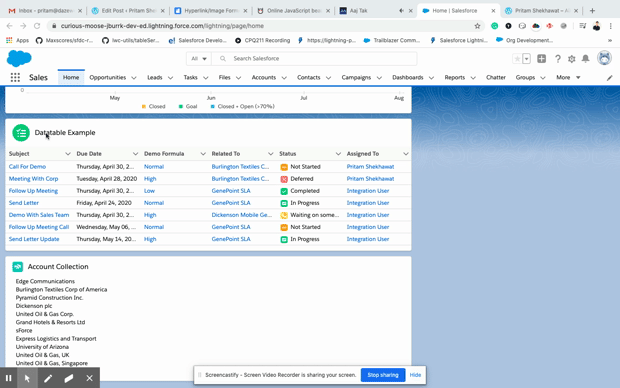